Not the snappiest title, I know, but it does describe perfectly what this post is about. I have been looking into credentials using PowerShell for some scripts I am writing.
I’m sure you will all have needed to include credentials in your scripts at sometime other, particularly if your scripts are creating resources in Azure. Using the following command lets you put credentials into an array for easy use within your script.
$Cred = Get-Credential
This command displays a dialog box where you can enter a username and password as shown below.
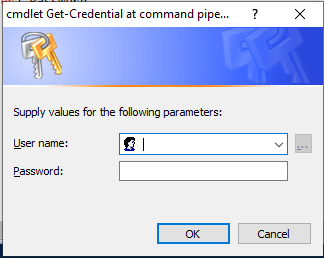
These details are then stored in the Array so that you can use them when required. You can even prepopulate the User name field by using the -Credential switch.
$Cred = Get-Credential -Credential Domain\User01
But what if I don’t want a prompt?
The above dialog box is an easy and safe way to add credentials to a script, but what if your script is designed not to be interactive?
Well, instead of using Get-Credential we can use the PSCREDENTIAL object directly.
First of all we need to create an encrypted password by doing the following.
$Password = ConvertTo-SecureString "Password01" -AsPlainText -Force
We can then create the new PSCREDENTIAL object
$cred = New-Object System.Management.Automation.PSCredential ("User01", $Password)
Avoiding storing password within the script
The problem with this approach is that the password is showing within the script, in plain text. So anyone with access to the script can get access to the password.
To get around this problem the password needs to be stored outside of the script, preferably in a way so that the user running the script can’t see the password. Within Azure this can be done by using a Key Vault. A Key Vault is an object that is used to store information that needs to be kept secure. The following should be done outside of your script.
Create your key Vault.
New-AzKeyVault -Name "<your-unique-keyvault-name>" -ResourceGroupName "myResourceGroup" -Location "UK South"
Create your password and store it in the Key Vault.
$Password = ConvertTo-SecureString "Password01" -AsPlainText -Force
Set-AzKeyVaultSecret -VaultName "<your-unique-keyvault-name>" -Name "MyPassword" -SecretValue $Password
Then, within your script get the password and create the credential.
$LocalPassword = Get-AzKeyVaultSecret -VaultName "<your-unique-keyvault-name>" -Name "MyPassword"
$Cred = New-Object System.Management.Automation.PSCredential ("User01", $LocalPassword)
If you want to hide the user name of the credential as well simply add an additional secret to the Key Vault and set it within your script in the same way as was done for the password.